How to use processing: The basics
- Karla G
- 27 mar 2015
- 3 Min. de lectura
In this post we will learn a little bit about the programming of computer graphics.
In processing you can get to do something like this:

Or even like this:

But for now, we have to start from the easiest forms. In this tutorial we will draw a pyramid in a 3D plane. The first thing you have to do in processing is opening the setup.
void setup(){
}
Inside the setup we must call the variables that will be used in different methods and also set the configurations of the processing output window.
The size of the window:
size(500,500,P3D);
500 is the number of pixels that the window will have of height and width. The term "P3D" defines that the window will have a 3D plane with 3D figures. There will be a x,y and z axis.
The camera:
For setting the camera, we have this variables:
float fov = PI/3.0;
// This is a variable of type float called fov. It has a value of PI/3.0;
float cameraZ = (height/2.0) / tan(fov/2.0);
// The variable cameraZ depends on the height of the window
What is perspective?
"Sets a perspective projection applying foreshortening, making distant objects appear smaller than closer ones. The parameters define a viewing volume with the shape of truncated pyramid. Objects near to the front of the volume appear their actual size, while farther objects appear smaller. This projection simulates the perspective of the world more accurately than orthographic projection."
This is the specific meaning found in processing.org.
Now that you know this, you can imagine what the next line does:
perspective(fov, float(width)/float(height), cameraZ/100.0, cameraZ*10.0);
// The perspective will depend in the width and height of the window.
Now we have a setup that looks like this:
void setup(){
float fov=PI/3.0;
float cameraZ= (height/2.0) / tan(fov/2.0);
perspective(fov, float(width)/float(height), cameraZ/100.0, cameraZ*10.0);
}
Now, we create another method, called draw. In this method of type void, the drawings will be set and created.
void draw(){
}
As you can see in the bottom pictures, processing is based in lines and TRIANGLES. This triangles are like the basic unit of the drawings that are made in processing with a 3D plane.
Inside the void draw() a color for the background will be set:
background(0,0,0); //Black
Then the camera will be called to the method.
camera(50*mouseX/500.0, -50*mouseY/500.0,40, 0,0,0, 0,1,0);
// This sets the mobility of the camera with the mouse over the axis X and Y
Now the interesting part, drawing:
beginShape(TRIANGLES);
//Now you can draw triangles
How can you draw them? Easy:
In order to do a figure it has to be separated in triangles. You have to know how many triangles and vertices a figure has.
After separating the figure in triangles, you have to locate each vertex of each triangle.
The triangles will be drawn vertex by vertex in groups of three (for each vertex of a triangle)
Here is a pyramid:
vertex(25,0,0);
vertex(12.5,0,12.5);
vertex(0,0,0);
vertex(0,0,0);
vertex(12.5,0,12.5);
vertex(12.5,20,6.25);
vertex(12.5,0,12.5);
vertex(25,0,0);
vertex(12.5,20,6.25);
vertex(25,0,0);
vertex(0,0,0);
vertex(12.5,20,6.25);
Finally you just have to end the shape of triangles:
endShape();
Now, this is how the void draw looks completely:
void draw(){
background(0,0,0);
camera(50*mouseX/500.0, -50*mouseY/500.0,40, 0,0,0, 0,1,0);
noFill();
stroke(255,255,255);
beginShape(TRIANGLES);
vertex(25,0,0);
vertex(12.5,0,12.5);
vertex(0,0,0);
vertex(0,0,0);
vertex(12.5,0,12.5);
vertex(12.5,20,6.25);
vertex(12.5,0,12.5);
vertex(25,0,0);
vertex(12.5,20,6.25);
vertex(25,0,0);
vertex(0,0,0);
vertex(12.5,20,6.25);
endShape();
}
My final product:
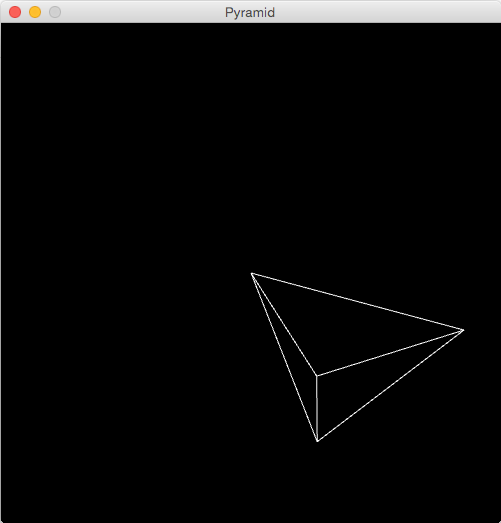
References:
Processing. (2001). Processing. 24 Marzo 2015, de Processing.org Sitio web: https://processing.org/
Comments